A quick visual reference for font sizes
Edit – Updated 2023 with new sizes.
Font Sizes marked with * require iOS 14
All sizes based on default dynamic type settings.
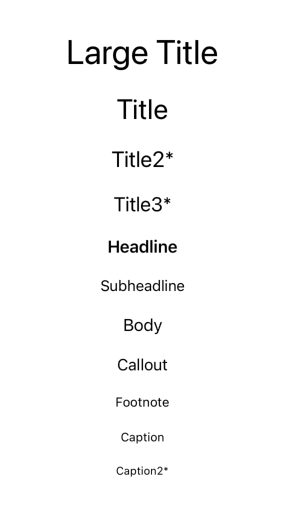
A quick visual reference for the standard SwiftUI button Styles:
To my surprise, one of VLC Remote’s users showed me how you actually can run multiple copies of VLC and remote control them with VLC Remote. (thank you Calvin!)
He is using them to stream to 4 zones in his house, but there are plenty of other reasons you might want to do this.
The trick is simply to run VLC from the command line and specify a different http-port value for each.
For Mac os, I used:
/Applications/VLC.app/Contents/MacOS/VLC --http-port 8090
/Applications/VLC.app/Contents/MacOS/VLC --http-port 8091
for Windows, the equivalent would be:
c:\Program Files (X86)\VideoLAN\VLC\VLC -VVV --http-port 8090
c:\Program Files (X86)\VideoLAN\VLC\VLC -VVV --http-port 8091
You’ll then need to add computers manually in VLC remote using the IP address of your computer and port 8090 (or whatever port you use).
If you’re doing this regularly, you’ll probably want to set up a batch file to start up your multiple instances. Calvin was able to set up his batch script so it pointed to individual audio devices in his streaming setup.
cd "c:\Program Files (X86)\VideoLAN\VLC\"
rem VLC session 1 with gigaport1 audio, port 8310, and russound1$
start cmd.exe /k "VLC -VVV --http-port 8310 --directx-audio-device={32776569-ABD9-4C9D-89B1-11E47A93EDEF} --directx-audio-speaker=Stereo \\atomic\russound1$"
rem VLc session 2 with gigaport2 audio, port 8320, and russound2$
start cmd.exe /k "VLC -VVV --http-port 8320 --directx-audio-device={39E33C1B-A451-4185-B470-2CBAEB769018} --directx-audio-speaker=Stereo \\atomic\russound2$"
rem VLC session 3 with gigaport3 audio, port 8330, and russound3$
start cmd.exe /k "VLC -VVV --http-port 8330 --directx-audio-device={CF04DBB5-952D-4AEE-80F6-AEC2AFDAF671} --direct x-audio-speaker=Stereo \\atomic\russound3$
rem vlc session 4 with gigaport4 audio, port 8340, and russound4$
start cmd.exe /k "VLC -VVV --http-port 8340 --directx-audio-device={EEF16A7C-842C-4DA3-BA65-E8F24C0A0266} --direct x-audio-speaker=Stereo \\atomic\russound4$
Back in 2014, I wrote about how Dolby were requiring me to remove ac3 support from VLC Streamer for somewhat questionable legal reasons
I included the email I received from their representative about the price I would have to pay
You should also know that there are fees associated with becoming licensed. Specifically, there is a $25,000 one-time initial fee and a recurring $5,000 annual maintenance fee. There is also a per-unit royalty that has a tiered structure, due quarterly, based on annual total usage, as follows:
0-100,000 downloads at $0.99 per download
100,001-1,000,000 downloads at $0.67 per download
1,000,001+ downloads at $0.45 per download
I was interested to get a call from a lawyer working for Adobe recently. They were being sued by Dolby for something related to these patents.
Bizarrely, Dolby were arguing that their pricing levels were so super secret that they couldn’t even disclose them in court.
Adobe found my old post and argued that if they were published on the web – they couldn’t be that secret.
I don’t have any details about the case, but I know they settled and it went away. Hopefully my pricing info made the difference…
Apple provides dynamic colours which match the system.
I couldn’t find a chart showing what they look like in light and dark mode, so here it is.
Dynamic Colours – light mode on the left – dark mode on the right
Codable is a kind of magic.
@Published certainly behaves like magic.
But if you try to add a @Published property to your codeable, then XCode complains
This one had me frustrated for many hours. The issue is that the encoder gets an instance of Published<Type> to encode. As far as I know there isn’t a proper way to get the actual value.
(normally, your code will call the getter on wrappedValue, but I’m not aware of a way to call that directly with Published)
In the end, the only solution I found was to Mirror the struct and extract the value from the ‘value’ Mirror.Child
This has been pretty typical of my SwiftUI experience. There is lots of magical functionality that falls out for minimal effort in a magical way.
But then you try to push the edges, act like a programmer and extend things – and you find that the basic API seems designed to frustrate you. Why would the Published struct not have a simple way to access the wrapped variable?
Anyway, for now at least – we can hack around the limitations
import Foundation import SwiftUI extension Published:Encodable where Value:Decodable { public func encode(to encoder: Encoder) throws { let mirror = Mirror(reflecting: self) if let valueChild = mirror.children.first(where: { (child) -> Bool in child.label == "value" }) { if let value = valueChild.value as? Encodable { do { try value.encode(to: encoder) return } catch let error { assertionFailure("Failed encoding: \(self) - \(error)") } } else { assertionFailure("Decodable Value not decodable. Odd \(self)") } } else { assertionFailure("Mirror Mirror on the wall - why no value y'all : \(self)") } } } extension Published:Decodable where Value:Decodable { public init(from decoder: Decoder) throws { self = Published(initialValue:try Value(from:decoder)) } }
tl;dr – UIApplication.shared.idleTimerDisabled now causes an undocumented crash if you compile with the iOS 13 SDK and use it from a background thread.
VLC Streamer is one of my oldest apps. It has been going strong since 2008!
It’s a pretty complex app with a bunch of different code paths and edge cases. It’s mostly written in Objective-C.
I uploaded a new build on Jun 15, 2019 to deal with some details around new device screens. No problems there.
I uploaded again on November 20th. There were two relevant changes.
I woke up on the 22nd to get loads of crash reports. One of my code paths calls this from a background thread:
[[UIApplication sharedApplication] setIdleTimerDisabled:YES];
This now causes a crash.
Three things of note here for me
Of course – better testing would have caught this, but how many of us really have proper UI testing in all of our code paths…
Update:
Building a test app with iOS 9 as the target still gives the crash.
Presumably this means that the change has come from using XCode 11.2.1 and the iOS 13 SDK.
I was very surprised when I downloaded ‘Ruler on Call EZ‘ from the Mac App store today only to find that it won’t run.
The issue is that it is a 32 bit app, and I have updated to Catalina.
It’s ridiculous that Apple is not just showing me this app, but even letting me download it.
It’s even more ridiculous that the ‘Information’ section says ‘Works on this iMac’
In the last week or so, both Apple and Google informed me that one of my apps was in a small way breaching store guidelines.
I’ll paraphrase both messages here:
One of your apps hasn’t been updated in years. It doesn’t meet current guidelines. If you don’t update it – we’ll remove it in 30 days.
Apple
and
One of your apps breaks the metadata guidelines. It has a quote in the app description.
We have removed it from the store.
Google
Both complaints are probably valid. The iOS app hasn’t been updated since 2015 and hasn’t been optimised for the latest devices. I’ll update it this month.
The Android app did have the following quote in the store description
VLC Remote is the latest Android app to earn my favor, and it’s a beauty
Androidnica
The quote has been there for at least 5 years. I’m not entirely sure whether it should count as a ‘user testimonial’ (banned) or a ‘third party review’ (I think those are ok). But either way – I’m happy to remove it.
Removing my app from the store just seems like a Massive Overreaction when they could simply have emailed me to request a change.
Every now and again – Apple demands my attention with a red dot on the System Preferences in the dock
When they do – I launch the preferences app, and my eye is immediately drawn to the icon at the top right on the ‘Notifications’ icon
Of course – that red dot isn’t a notification dot. That’s an icon of a notification dot – not an actual notification dot
I’m supposed to ignore that one, and look for the second one on the ‘software update’ icon
That’s just bad UI
Apple have fixed this as of Mac OS 10.15